How to Tail Docker Logs - Detailed Guide
Managing Docker container logs is essential for debugging and monitoring application performance. Tailoring Docker logs allows for real-time insights, quick issue resolution, and optimized performance. This guide focuses on efficient methods for tailing Docker logs, with clear examples and command options to streamline log management.
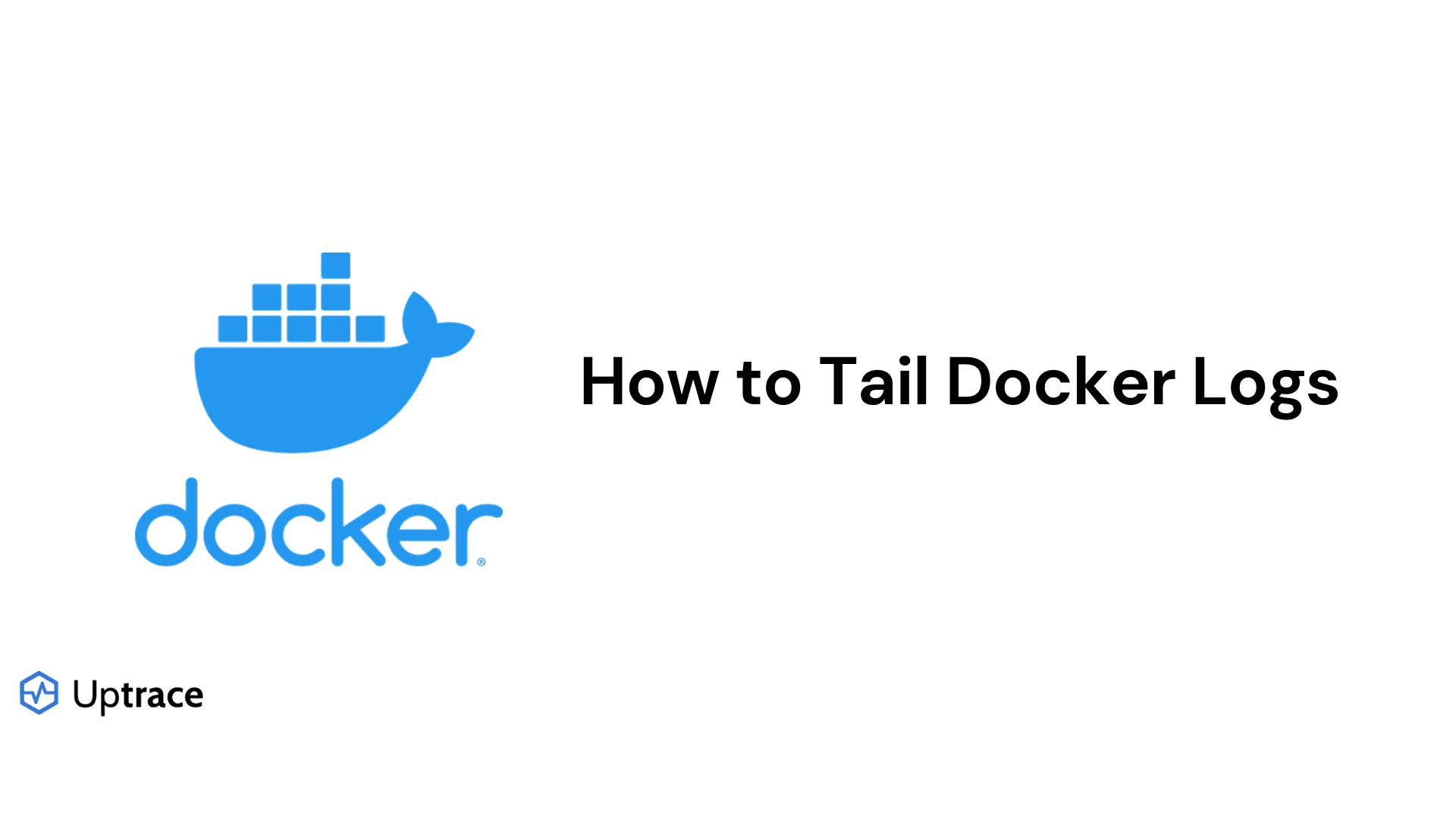
What are Docker Logs?
Docker logs are important records that capture the activities and events generated by Docker containers while running. These logs provide crucial information about the performance and behavior of containerized applications, which is incredibly useful for developers and system administrators when it comes to diagnosing problems, monitoring the applications, and resolving issues. Docker logs collect and store the output produced by applications within the containers, such as standard output (stdout) and standard error (stderr), offering comprehensive visibility into the runtime behavior of the containers and their applications.
Key Features of Docker Logs
- Output of Containerized Applications: Logs capture everything written to the stdout and stderr streams by the applications running in the container.
- Real-Time Monitoring: You can view logs as they are generated using the
docker logs --follow
command, allowing you to track live outputs from running containers. - Log Retention: Docker maintains log history, which can be queried using specific commands. However, the size of logs can grow significantly over time, requiring strategies like log rotation to manage disk usage.
- Multiple Log Drivers: Docker supports different log drivers that determine how logs are stored and managed. For example, logs can be sent to a logging service (e.g., Syslog, Fluentd, or journald) rather than simply being stored on disk.
Why Are Docker Logs Important?
- Debugging: Logs provide detailed information about errors and issues within applications, helping developers trace the root cause of problems.
- Monitoring: Logs help in tracking application performance and identifying unexpected behaviors.
- Compliance and Auditing: Logs are often used for compliance, as they provide a record of activity that can be reviewed in the future.
Key Docker Log Commands
docker logs
The primary command for accessing Docker logs is docker logs
. This command allows you to view the logs of a specific container.
Basic Usage
docker logs [OPTIONS] CONTAINER
- CONTAINER: The name or ID of the container.
Key Options
--tail
: Shows the last N lines of logs.--since
: Filters logs based on time.--follow
: Streams logs in real-time.--timestamps
: Adds timestamps to log output.--head
: View the first N lines of logs.
Examples
To view the last 100 lines of logs:
docker logs --tail 100 CONTAINER_NAME
To follow logs in real-time:
docker logs --follow CONTAINER_NAME
To view the first 100 lines of logs:
docker logs --head 100 CONTAINER_NAME
To view logs from the last hour:
docker logs --since 1h CONTAINER_NAME
docker-compose logs
If you're using Docker Compose, you can view logs for multiple services at once.
Basic Usage
docker-compose logs [OPTIONS] [SERVICE...]
Key Options
--tail
: Limits output to the last N lines.--follow
: Streams logs for all specified services.--timestamps
: Includes timestamps in log output.
Examples
To tail logs for a specific service:
docker-compose logs --tail 100 SERVICE_NAME
To follow logs:
docker-compose logs --follow
Advanced Log Management
Filtering and Searching Logs
Using grep
with Docker logs can help in filtering and searching specific entries.
Examples
To search for a keyword in logs:
docker logs CONTAINER_NAME | grep "KEYWORD"
To view logs for a specific time period:
docker logs --since "2023-01-01T00:00:00" CONTAINER_NAME
Exporting Logs to a File
Exporting logs to a file allows for offline analysis and long-term storage.
Examples
To pipe logs to a file:
docker logs CONTAINER_NAME > logs.txt
To append Docker logs to a file and watch them in real-time:
docker logs --follow CONTAINER_NAME | tee logs.txt
Clearing and Truncating Docker Logs
Docker doesn't provide a direct command to clear logs, but there are methods to truncate log files or use log rotation strategies.
Clear logs by restarting the container:
docker restart CONTAINER_NAME
Truncate logs:
truncate -s 0 /var/lib/docker/containers/CONTAINER_ID/CONTAINER_ID-json.log
Viewing Logs with Timestamps
Adding timestamps to logs can provide context and timing information for your log entries.
docker logs --timestamps CONTAINER_NAME
Monitoring Logs in Real-Time with watch
The watch
command allows you to monitor changes to Docker logs in real-time by repeatedly executing a command.
watch docker logs CONTAINER_NAME
Kubernetes Log Management
In Kubernetes, managing logs involves different commands.
kubectl logs
To view logs of a pod in Kubernetes:
Mastering Kubernetes Logging - Detailed Guide to kubectl logs
Examples
To view the last 100 lines:
kubectl logs POD_NAME --tail=100
To follow logs:
kubectl logs POD_NAME --follow
To view the first 100 lines:
kubectl logs POD_NAME --head=100
Best Practices for Managing Docker Logs
Effective management of Docker logs is crucial for maintaining system performance and facilitating efficient troubleshooting. Here are some best practices to optimize your Docker log management:
Log Rotation and Size Management
For large-scale applications, it's essential to manage log sizes effectively to prevent them from consuming excessive disk space.
To enable log rotation in Docker:
docker run --log-opt max-size=10m --log-opt max-file=3 CONTAINER_NAME
This configuration limits logs to 10 MB and retains up to 3 log files. You can adjust these values based on your specific needs and storage capacity.
Use Appropriate Logging Drivers
Docker supports various logging drivers. Choose the one that best fits your infrastructure and requirements:
docker run --log-driver json-file --log-opt max-size=10m --log-opt max-file=3 CONTAINER_NAME
Popular options include:
- json-file (default)
- syslog
- journald
- fluentd
Implement Centralized Logging
For multi-container applications or distributed systems, consider implementing a centralized logging solution:
- Use ELK stack (Elasticsearch, Logstash, Kibana)
- Implement cloud-based solutions like AWS CloudWatch or Google Cloud Logging
- Utilize specialized log management tools like Splunk or Sumo Logic
Structure Your Logs
Encourage developers to use structured logging formats (e.g., JSON) within applications:
import json
import logging
logging.basicConfig(format='%(message)s')
logger = logging.getLogger()
log_data = {
'level': 'INFO',
'message': 'User logged in',
'user_id': 12345
}
logger.info(json.dumps(log_data))
This practice makes logs more easily parseable and searchable.
Monitor Log Volume and Set Alerts
Implement monitoring for log volume and set up alerts for unusual spikes:
- Use monitoring tools like Prometheus with Grafana
- Set up alerts for rapid increases in log volume, which might indicate issues
Configuring Prometheus Rules
First, create a Prometheus rule to detect rapid increases in log volume. Add the following to your Prometheus rules file (e.g., alert_rules.yml
):
groups:
- name: log_volume_alerts
rules:
- alert: LogVolumeSpike
expr: rate(container_log_bytes_total[5m]) > 1024 * 1024 * 10 # More than 10MB/s over 5 minutes
for: 2m
labels:
severity: warning
annotations:
summary: 'High log volume detected for container {{ $labels.container_name }}'
description: 'Container {{ $labels.container_name }} is logging at a rate of {{ $value | humanize }}B/s for the last 2 minutes.'
This rule triggers an alert when the log volume rate exceeds 10MB/s over a 5-minute period, sustained for 2 minutes.
Configuring Alertmanager
Next, configure Alertmanager to send notifications for these alerts. Here's an example configuration (alertmanager.yml
) that sends alerts via email and Slack:
global:
smtp_smarthost: 'smtp.example.com:587'
smtp_from: 'alertmanager@example.com'
smtp_auth_username: 'username'
smtp_auth_password: 'password'
route:
group_by: ['alertname']
receiver: 'team-emails'
routes:
- match:
severity: warning
receiver: 'team-slack'
receivers:
- name: 'team-emails'
email_configs:
- to: 'team@example.com'
- name: 'team-slack'
slack_configs:
- api_url: 'https://hooks.slack.com/services/T00000000/B00000000/XXXXXXXXXXXXXXXXXXXXXXXX'
channel: '#alerts'
text: "{{ range .Alerts }}{{ .Annotations.description }}\n{{ end }}"
This configuration sends all alerts to the team email, and warning-level alerts (like our LogVolumeSpike) to a Slack channel.
Implementing the Alert System
Save these configurations in your Prometheus and Alertmanager configuration directories.
Restart Prometheus and Alertmanager to apply the changes:
sudo systemctl restart prometheus sudo systemctl restart alertmanager
Verify that the rules are loaded in Prometheus by checking the "Rules" page in the Prometheus web interface.
Responding to Alerts
When you receive an alert about a log volume spike:
- Investigate the affected container immediately.
- Check the application logs for error messages or unusual activity.
- Monitor system resources to ensure the high log volume isn't impacting performance.
- If necessary, adjust log levels or implement rate limiting for logs.
- Consider implementing log sampling for high-volume events to reduce overall log volume while still capturing important information.
By setting up these alerts, you can quickly identify and respond to potential issues indicated by unusual increases in log volume, helping to maintain the stability and performance of your Docker environments.
Regularly Review and Clean Up Logs
Establish a routine for reviewing and cleaning up old logs:
- Automate the process of archiving old logs to cold storage
- Regularly review log retention policies and adjust as needed
Here are some code examples to implement these practices:
1. Automating Log Archival
Create a bash script to automatically archive old logs to a separate storage location. This script compresses logs older than 30 days and moves them to an archive directory:
#!/bin/bash
# Define variables
LOG_DIR="/var/lib/docker/containers"
ARCHIVE_DIR="/path/to/log/archive"
DAYS_TO_KEEP=30
# Create archive directory if it doesn't exist
mkdir -p $ARCHIVE_DIR
# Find and compress log files older than 30 days
find $LOG_DIR -name "*.log" -type f -mtime +$DAYS_TO_KEEP | while read file
do
gzip "$file"
mv "${file}.gz" $ARCHIVE_DIR
done
# Optional: Upload to cold storage (e.g., AWS S3)
# Uncomment and configure AWS CLI for this to work
# aws s3 sync $ARCHIVE_DIR s3://your-bucket-name/docker-logs/
Save this script as archive_docker_logs.sh
, make it executable with chmod +x archive_docker_logs.sh
, and set up a cron job to run it regularly:
# Add this line to /etc/crontab to run the script daily at 1 AM
0 1 * * * root /path/to/archive_docker_logs.sh
2. Managing Log Retention Policies
For Docker, you can manage log retention by configuring the logging driver. Here's an example of how to set up log rotation and retention in your Docker daemon configuration file (/etc/docker/daemon.json
):
{
"log-driver": "json-file",
"log-opts": {
"max-size": "10m",
"max-file": "3",
"compress": "true"
}
}
This configuration:
- Limits each log file to 10 MB
- Keeps a maximum of 3 log files
- Compresses older log files
For Kubernetes, you can use a ConfigMap
to manage log retention policies across your cluster. Here's an example:
apiVersion: v1
kind: ConfigMap
metadata:
name: fluentd-config
data:
fluent.conf: |
<match **>
@type file
path /var/log/fluent/myapp
compress gzip
<buffer>
timekey 1d
timekey_use_utc true
timekey_wait 10m
</buffer>
<format>
@type json
</format>
</match>
This Fluentd configuration:
- Collects logs from all sources
- Stores them in daily log files
- Compresses the logs using gzip
- Rotates logs daily
To review and adjust these policies:
- Set up monitoring alerts for log volume and storage usage.
- Regularly audit your log data to ensure you're capturing necessary information without over-logging.
- Review compliance requirements and adjust retention periods accordingly.
- Consider implementing a log analysis tool to help identify patterns and optimize your logging strategy.
Secure Your Logs
Ensure that your logs are secure and comply with relevant regulations:
- Encrypt logs in transit and at rest
- Implement access controls to restrict who can view logs
- Ensure compliance with regulations like GDPR or HIPAA if applicable
Here are some code examples to implement these security measures:
1. Encrypting Logs
For encrypting logs at rest, you can use tools like logrotate
with GPG encryption:
# /etc/logrotate.d/docker-container-logs
/var/lib/docker/containers/*/*.log {
rotate 7
daily
compress
missingok
notifempty
postrotate
for f in /var/lib/docker/containers/*/*.log; do
gpg --encrypt --recipient your@email.com -o "$f.gpg" "$f"
rm "$f"
done
endscript
}
For encrypting logs in transit, use TLS when sending logs to a remote server. Here's an example using rsyslog
:
# /etc/rsyslog.d/docker.conf
$DefaultNetstreamDriver gtls
$DefaultNetstreamDriverCAFile /path/to/ca.pem
$DefaultNetstreamDriverCertFile /path/to/cert.pem
$DefaultNetstreamDriverKeyFile /path/to/key.pem
$ActionSendStreamDriver gtls
$ActionSendStreamDriverMode 1
$ActionSendStreamDriverAuthMode x509/name
$ActionSendStreamDriverPermittedPeer central-log-server.example.com
*.* @@central-log-server.example.com:6514
2. Implementing Access Controls
Use Linux file permissions and ACLs to restrict access to log files:
# Set appropriate ownership and permissions
sudo chown root:syslog /var/lib/docker/containers/*/*.log
sudo chmod 640 /var/lib/docker/containers/*/*.log
# Use ACLs for more granular control
sudo setfacl -m u:specific_user:r /var/lib/docker/containers/*/*.log
For Kubernetes, use Role-Based Access Control (RBAC):
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: log-reader
rules:
- apiGroups: ['']
resources: ['pods', 'pods/log']
verbs: ['get', 'list']
---
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: log-reader-binding
namespace: default
subjects:
- kind: User
name: jane
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: log-reader
apiGroup: rbac.authorization.k8s.io
3. Ensuring Compliance (GDPR/HIPAA)
For GDPR compliance, implement a log retention policy and ensure personal data is pseudonymized:
import re
import hashlib
def pseudonymize_log(log_line):
# Pseudonymize email addresses
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
log_line = re.sub(email_pattern, lambda m: hashlib.sha256(m.group(0).encode()).hexdigest(), log_line)
# Pseudonymize IP addresses
ip_pattern = r'\b(?:\d{1,3}\.){3}\d{1,3}\b'
log_line = re.sub(ip_pattern, lambda m: hashlib.sha256(m.group(0).encode()).hexdigest(), log_line)
return log_line
# Example usage
original_log = "User email@example.com logged in from 192.168.1.1"
pseudonymized_log = pseudonymize_log(original_log)
print(pseudonymized_log)
For HIPAA compliance, ensure all Protected Health Information (PHI) is encrypted and implement strict access controls as shown in the previous examples.
Summary
Efficient log management is critical for maintaining Docker containers and applications. By utilizing commands like docker logs
, docker-compose logs
, and kubectl logs
, you can monitor real-time outputs, troubleshoot issues, and analyze historical data. Incorporating these methods into your workflow will enhance your ability to manage and maintain your Docker environments effectively.
How Uptrace Integrates with Docker Logs
Uptrace provides advanced observability and monitoring logs features that integrate seamlessly with Docker. By leveraging Uptrace's capabilities, you can enhance your log management strategies, gain deeper insights into container performance, and ensure the reliability of your applications.
FAQ
How do I view real-time logs for a Docker container?
To view real-time logs, use the --follow
or -f
option with the docker logs
command:
docker logs --follow CONTAINER_NAME
Can I see logs from multiple Docker containers at once?
Yes, if you're using Docker Compose, you can view logs from multiple services simultaneously:
docker-compose logs --follow
How can I limit the amount of log output I see?
Use the --tail
option to limit the number of lines:
docker logs --tail 100 CONTAINER_NAME
Is it possible to view logs from a specific time range?
Yes, use the --since
option:
docker logs --since "2023-01-01T00:00:00" CONTAINER_NAME
How do I clear Docker logs without removing the container?
You can truncate the log file:
truncate -s 0 /var/lib/docker/containers/CONTAINER_ID/CONTAINER_ID-json.log
Can I export Docker logs to a file?
Yes, you can redirect the output to a file:
docker logs CONTAINER_NAME > logs.txt
You may also be interested in: