How to Fix "Upstream Connect Error" in 7 Different Contexts
The error "upstream connect error or disconnect/reset before headers. reset reason: connection failure" has become a challenge for DevOps teams. This critical error, occurring when services fail to establish or maintain connections with their upstream dependencies, can significantly impact system reliability and user experience. While this error is most commonly associated with Nginx and proxy servers, it can manifest across various environments including Kubernetes, Docker containers, cloud services, and monitoring systems. This guide provides detailed solutions and prevention strategies across seven different contexts, helping teams quickly identify root causes, implement effective fixes, and prevent future occurrences of these connection failures.
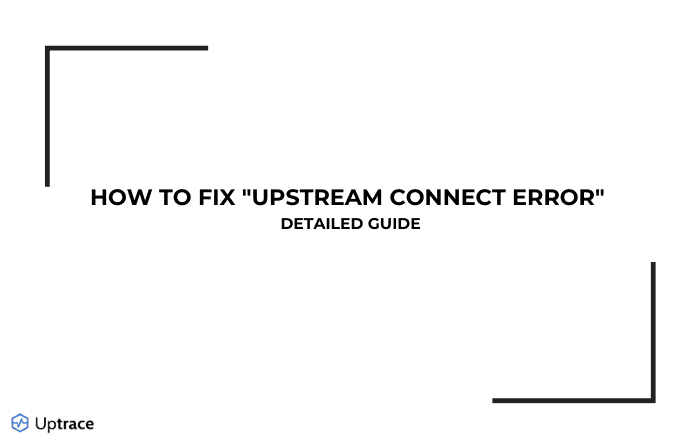
Introduction
The error message "upstream connect error or disconnect/reset before headers. reset reason: connection failure" is one of the most common issues DevOps engineers encounter in modern distributed systems. This error typically occurs when one service fails to establish or maintain a connection with an upstream service it depends on, often due to network issues, misconfiguration, or service unavailability. While initially appearing in Nginx logs, similar connection failures can manifest across various modern infrastructure components, making it crucial for DevOps teams to understand how to diagnose and fix these errors across different contexts.
This connection failure can be particularly challenging to troubleshoot because it can stem from multiple sources: the upstream service might be down, network connectivity could be interrupted, security groups might be misconfigured, or SSL certificates might be faulty. Depending on the affected components and your architecture's resilience, the error's impact can range from minor service degradation to a complete system outage.
Error Patterns Overview
Error Message | Common Cause | Environment | Severity |
---|---|---|---|
upstream connect error or disconnect/reset before headers | Network connectivity | Nginx/Proxy | High |
Connection refused | Service unavailable | General | High |
Connection timed out | Slow response | Load Balancers | Medium |
no healthy upstream | Failed health checks | Service Mesh | Critical |
Browser and Client Applications Error Manifestation
When an upstream connect error occurs, different browsers and client applications may display the error in various ways to end users. Understanding these manifestations can help in faster problem identification and better user communication.
Browser Error Displays
Browser | Error Display | User Message | HTTP Status |
---|---|---|---|
Chrome | ERR_CONNECTION_REFUSED | This site can't be reached. Website refused to connect. | 502 |
Firefox | Unable to connect | The connection was reset. Website may be temporarily unavailable or too busy. | 502 |
Safari | Safari Can't Connect to the Server | Safari can't open the page because the server unexpectedly dropped the connection. | 502 |
Edge | Can't reach this page | Website took too long to respond | 502 |
Mobile Chrome | Connection Reset | The site can't be reached. The connection was reset. | 502 |
Mobile Safari | Safari Cannot Open the Page | A connection to the server could not be established. | 502 |
Client Applications Behavior
REST Clients
Client | Error Manifestation | Additional Info |
---|---|---|
cURL | curl: (56) Recv failure: Connection reset by peer | Shows detailed error with verbose flag (-v) |
Postman | Could not get response | Displays detailed timing and request info |
Insomnia | Failed to connect | Shows full request/response cycle |
Axios | ECONNREFUSED | Includes error code and stack trace |
Fetch API | TypeError: Failed to fetch | Network error in console |
Mobile Applications
Platform | Common Display | User Experience Impact |
---|---|---|
iOS Native | "Connection Error" | App might show retry button |
Android Native | "Unable to connect to server" | May trigger automatic retry |
React Native | "Network request failed" | Generic error handling |
Flutter | "SocketException" | Platform-specific error handling |
Troubleshooting Tips
Browser-specific Investigation
// Browser console check fetch('https://api.example.com') .then((response) => response.json()) .catch((error) => console.error('Error:', error))
Client Application Debugging
# cURL verbose mode curl -v https://api.example.com
Mobile Application Analysis
// Android logging Log.e("NetworkError", "Upstream connection failed", exception);
Best Practices for Error Handling
Implement User-Friendly Error Messages
try { const response = await fetch(url) } catch (error) { if (error.name === 'TypeError' && error.message === 'Failed to fetch') { showUserFriendlyError('Service temporarily unavailable. Please try again later.') } }
Add Retry Logic
const fetchWithRetry = async (url, retries = 3) => { for (let i = 0; i < retries; i++) { try { return await fetch(url) } catch (error) { if (i === retries - 1) throw error await new Promise((resolve) => setTimeout(resolve, 1000 * Math.pow(2, i))) } } }
1. Nginx and Reverse Proxies
Symptoms
Error Message | Possible Cause | Severity |
---|---|---|
502 Bad Gateway | Backend server down | Critical |
504 Gateway Timeout | Backend slow response | High |
connect() failed | Network issues | High |
upstream timed out | Timeout configuration | Medium |
Diagnostic Steps
Check Nginx Status
systemctl status nginx nginx -t
Review Error Logs
tail -f /var/log/nginx/error.log
Verify Backend Availability
curl -I http://backend-server
Common Solutions
1. Backend Server Issues
upstream backend {
server backend1.example.com:8080 max_fails=3 fail_timeout=30s;
server backend2.example.com:8080 backup;
keepalive 32;
}
2. Timeout Configuration
server {
location / {
proxy_connect_timeout 60s;
proxy_send_timeout 60s;
proxy_read_timeout 60s;
proxy_next_upstream error timeout;
}
}
Prevention Measures
- Implement health checks
- Configure proper timeouts
- Set up monitoring
- Use backup servers
- Enable keepalive connections
2. Spring Boot and Microservices
Symptoms
Error Message | Possible Cause | Severity |
---|---|---|
Connection refused | Service down | Critical |
Load balancer error | Service discovery issue | High |
Circuit breaker open | Service degradation | Medium |
Timeout occurred | Slow response | Medium |
Diagnostic Steps
Check Service Status
curl -I http://service-name/actuator/health
Review Application Logs
tail -f application.log
Verify Service Discovery
curl -X GET http://eureka-server:8761/eureka/apps
Common Solutions
1. Service Discovery Issues
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka/
instance:
preferIpAddress: true
leaseRenewalIntervalInSeconds: 30
2. Circuit Breaker Configuration
@CircuitBreaker(name = "backendService", fallbackMethod = "fallbackMethod")
public String serviceCall() {
// Service call
}
public String fallbackMethod(Exception ex) {
return "Fallback Response";
}
Prevention Measures
- Implement circuit breakers
- Configure retry policies
- Set up fallback methods
- Enable service discovery
- Configure proper timeouts
3. Kubernetes Environment
Symptoms
Error Message | Possible Cause | Severity |
---|---|---|
Failed to establish connection | Pod networking issue | Critical |
Service unavailable | Service misconfiguration | High |
Connection timed out | Network policy blocking | Medium |
DNS resolution failed | CoreDNS issues | High |
Diagnostic Steps
Check Pod Status
kubectl get pods -n namespace kubectl describe pod pod-name
Review Service Configuration
kubectl get svc kubectl describe svc service-name
Verify Network Policies
kubectl get networkpolicies kubectl describe networkpolicy policy-name
Common Solutions
1. Pod Communication Issues
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-service-access
spec:
podSelector:
matchLabels:
app: frontend
ingress:
- from:
- podSelector:
matchLabels:
app: backend
2. Service Configuration
apiVersion: v1
kind: Service
metadata:
name: backend-service
spec:
selector:
app: backend
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: ClusterIP
Prevention Measures
- Implement readiness probes
- Configure liveness probes
- Set up network policies
- Use service mesh
- Monitor pod health
4. Docker and Container Networking
Symptoms
Error Message | Possible Cause | Severity |
---|---|---|
Network not found | Missing network configuration | Critical |
Container not found | DNS resolution issue | High |
Connection refused | Container not ready | Medium |
Network timeout | Network driver issue | High |
Diagnostic Steps
Check Network Status
docker network ls docker network inspect network-name
Verify Container Connectivity
docker exec container-name ping service-name docker logs container-name
Review Network Configuration
docker-compose config
Common Solutions
1. Network Configuration
version: '3'
services:
frontend:
networks:
- app-network
depends_on:
- backend
backend:
networks:
- app-network
healthcheck:
test: ['CMD', 'curl', '-f', 'http://localhost:8080/health']
interval: 30s
timeout: 10s
retries: 3
networks:
app-network:
driver: bridge
2. DNS Resolution
# Add custom DNS to daemon.json
{
"dns": ["8.8.8.8", "8.8.4.4"]
}
Prevention Measures
- Use proper network modes
- Configure DNS correctly
- Implement healthchecks
- Set container dependencies
- Monitor network performance
5. Cloud Services (AWS/Azure/GCP)
Symptoms
Error Message | Possible Cause | Severity |
---|---|---|
Security group blocking | Incorrect security rules | Critical |
Subnet connectivity | VPC configuration | High |
Load balancer error | Health check failure | High |
Cross-zone issue | Zone configuration | Medium |
Diagnostic Steps
Check Security Groups
aws ec2 describe-security-groups --group-ids sg-xxx
Verify VPC Configuration
aws ec2 describe-vpc-peering-connections
Review Load Balancer Status
aws elbv2 describe-target-health --target-group-arn arn:xxx
Common Solutions
1. Security Group Configuration
{
"GroupId": "sg-xxx",
"IpPermissions": [
{
"IpProtocol": "tcp",
"FromPort": 80,
"ToPort": 80,
"IpRanges": [{ "CidrIp": "10.0.0.0/16" }]
}
]
}
2. Load Balancer Health Checks
{
"HealthCheckProtocol": "HTTP",
"HealthCheckPort": "80",
"HealthCheckPath": "/health",
"HealthCheckIntervalSeconds": 30,
"HealthyThresholdCount": 2,
"UnhealthyThresholdCount": 3
}
Prevention Measures
- Regular security audit
- Proper VPC design
- Multi-zone deployment
- Automated health checks
- Monitoring and alerts
6. Proxy Servers and Load Balancers
Symptoms
Error Message | Possible Cause | Severity |
---|---|---|
no server available | All backends down | Critical |
connect() failed | Network connectivity | High |
connection timed out | Slow response | Medium |
proxy protocol error | Configuration issue | Medium |
Diagnostic Steps
Check Proxy Status
haproxy -c -f /etc/haproxy/haproxy.cfg systemctl status haproxy
Monitor Backend Status
echo "show stat" | socat stdio /var/run/haproxy.sock
Review Performance Metrics
tail -f /var/log/haproxy.log | grep "time="
Common Solutions
1. Backend Configuration
backend web-backend
option httpchk GET /health
http-check expect status 200
server web1 10.0.0.1:80 check
server web2 10.0.0.2:80 check backup
timeout connect 5s
timeout server 30s
2. SSL/TLS Configuration
frontend https-frontend
bind *:443 ssl crt /etc/ssl/certs/example.pem
mode http
option httplog
default_backend web-backend
Prevention Measures
- Regular health checks
- Backup servers
- Proper SSL configuration
- Load balancing strategy
- Monitoring system
7. Monitoring and Logging Systems
Symptoms
Error Message | Possible Cause | Severity |
---|---|---|
Metric collection failed | Prometheus scrape error | High |
Log shipping error | Filebeat configuration | Medium |
Connection refused | ELK stack issue | High |
Authentication failed | Incorrect credentials | Critical |
Diagnostic Steps
Check Monitoring Service
curl -I http://prometheus:9090/-/healthy systemctl status prometheus
Verify Log Collection
filebeat test config -c /etc/filebeat/filebeat.yml filebeat test output
Review Elasticsearch Status
curl -X GET "localhost:9200/_cluster/health"
Common Solutions
1. Prometheus Configuration
scrape_configs:
- job_name: 'upstream-monitor'
metrics_path: '/metrics'
static_configs:
- targets: ['app:8080']
scrape_interval: 15s
scrape_timeout: 10s
2. Logging Pipeline Setup
filebeat.inputs:
- type: log
enabled: true
paths:
- /var/log/upstream/*.log
fields:
service: upstream
output.elasticsearch:
hosts: ['elasticsearch:9200']
index: 'upstream-logs-%{+yyyy.MM.dd}'
Prevention Measures
- Regular metric validation
- Log rotation policy
- Storage capacity planning
- Alert configuration
- Backup logging pipeline
Conclusion
Quick Reference Table
Context | Primary Tool | Key Configuration | Common Fix |
---|---|---|---|
Nginx | nginx -t | nginx.conf | Restart service |
Spring Boot | actuator | application.yml | Circuit breaker |
Kubernetes | kubectl | NetworkPolicy | Network policy |
Docker | docker inspect | docker-compose.yml | Network config |
Cloud | AWS CLI | Security Groups | Update rules |
Proxy | haproxy | haproxy.cfg | Backend check |
Monitoring | prometheus | prometheus.yml | Scrape config |
Troubleshooting Flowchart
FAQ
How quickly can upstream connect errors be resolved? Most upstream connect errors can be resolved within minutes to hours, depending on the context:
- Simple configuration issues: 5-15 minutes
- Network-related problems: 15-60 minutes
- Complex distributed system issues: 1-4 hours
- Cloud infrastructure problems: 1-24 hours
Can upstream errors occur even with proper monitoring? Yes, upstream errors can still occur even with monitoring in place. However, good monitoring helps:
- Detect issues before they become critical
- Identify root causes faster
- Provide historical context for troubleshooting
- Enable proactive maintenance
Should I implement all prevention measures at once? No, it's recommended to implement prevention measures gradually:
- Start with basic monitoring
- Add health checks
- Implement circuit breakers
- Configure proper timeouts
- Add redundancy measures
How can I distinguish between different types of upstream errors? Look for specific patterns in error messages:
- "Connection refused": Service is down
- "Timeout": Service is slow
- "No route to host": Network issue
- "Certificate error": SSL/TLS problem
What are the minimum monitoring requirements? Essential monitoring components include:
- Basic health checks
- Response time monitoring
- Error rate tracking
- Resource utilization metrics
- Log aggregation
Can automated tools prevent upstream errors? Automated tools can help prevent some errors through:
- Automatic failover
- Self-healing mechanisms
- Predictive analytics
- Auto-scaling But they can't prevent all types of failures.
How do microservices affect upstream error handling? Microservices add complexity to error handling:
- More potential points of failure
- Complex dependency chains
- Distributed tracing requirements
- Service discovery challenges
You may also be interested in: