How to Set Up Prometheus for Docker [Step-by-Step Guide]
Prometheus is a go-to solution for monitoring containerized environments. This detailed guide will walk you through setting up Prometheus for Docker, helping you achieve robust monitoring for your containerized applications.
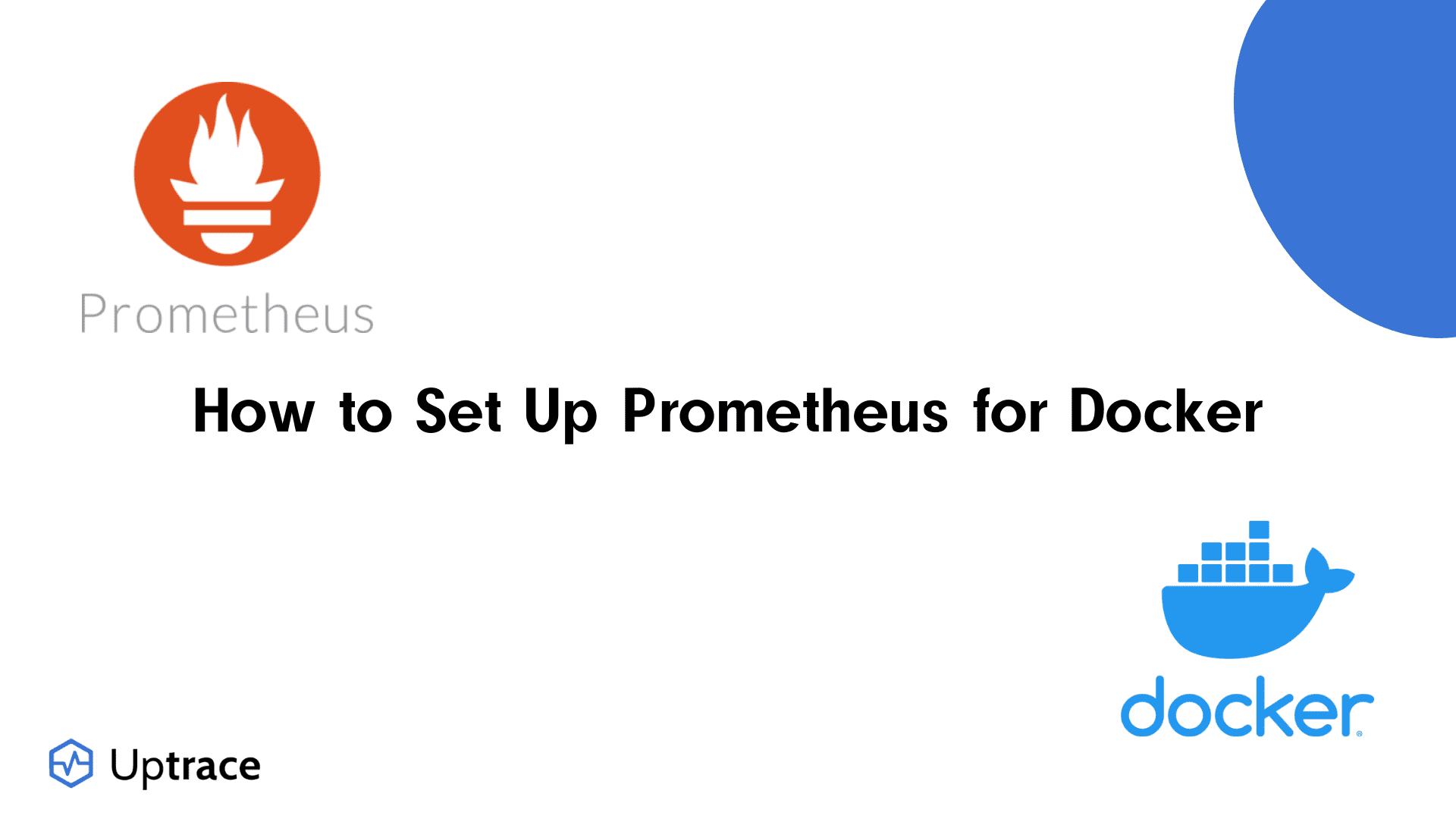
Introduction to Prometheus and Docker
Prometheus is an open-source monitoring and alerting toolkit, while Docker is a platform for developing, shipping, and running applications in containers. When combined, they provide a powerful solution for monitoring containerized applications.
Understanding Prometheus
Prometheus is a systems and service monitoring system. It collects metrics from configured targets at given intervals, evaluates rule expressions, displays the results, and can trigger alerts if some condition is observed to be true.
Key Features of Prometheus
- Multi-dimensional data model
- PromQL, a flexible query language
- Time series collection via a pull model over HTTP
- Pushing time series supported via an intermediary gateway
- Targets discovered via service discovery or static configuration
Docker Basics
Docker is a platform for containerizing applications. It allows you to package an application with all of its dependencies into a standardized unit for software development.
Key Concepts in Docker
- Containers: Lightweight, standalone, executable packages of software
- Images: Read-only templates used to create containers
- Dockerfile: A script of instructions used to create a Docker image
- Docker Compose: A tool for defining and running multi-container Docker applications
Integrating Prometheus with Docker
Integrating Prometheus with Docker allows you to monitor your containerized applications effectively. This integration provides several benefits:
- Easy deployment: Prometheus can be run as a Docker container, simplifying setup and management.
- Service discovery: Prometheus can automatically discover and monitor Docker containers.
- Resource efficiency: Both Prometheus and your applications can run in containers, optimizing resource usage.
Step-by-Step Setup of Prometheus for Docker
Let's start the process of setting up Prometheus for Docker monitoring:
- Create a Prometheus configuration file (
prometheus.yml
):
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'docker'
docker_sd_configs:
- host: unix:///var/run/docker.sock
relabel_configs:
- source_labels: [__meta_docker_container_name]
regex: '/(.*)'
target_label: container_name
replacement: '$1'
- Create a Docker network for Prometheus:
docker network create prometheus-network
- Run Prometheus as a Docker container:
docker run -d --name prometheus \
--network prometheus-network \
-p 9090:9090 \
-v /path/to/prometheus.yml:/etc/prometheus/prometheus.yml \
-v /var/run/docker.sock:/var/run/docker.sock \
prom/prometheus
Verify Prometheus is running:
- Open a web browser and navigate to
http://localhost:9090
- You should see the Prometheus web interface
- Open a web browser and navigate to
Configure your Docker containers for Prometheus scraping:
- Add the following labels to your Docker containers:
labels: - 'prometheus.io/scrape=true' - 'prometheus.io/port=<metrics-port>'
- Add the following labels to your Docker containers:
Restart your Docker containers with the new labels.
Check Prometheus targets:
- In the Prometheus web interface, go to Status > Targets
- You should see your Docker containers listed as targets
Best Practices for Using Prometheus with Docker
- Use Docker labels to configure scraping: Add labels to your containers to control how Prometheus scrapes them.
- Implement health checks: Use Docker health checks to ensure your containers are functioning correctly.
- Monitor Docker itself: Set up Prometheus to monitor Docker engine metrics.
- Use Grafana for visualization: Combine Prometheus with Grafana for powerful data visualization.
- Implement alerting: Use Prometheus Alertmanager to set up alerts for critical issues.
Using the Prometheus Docker Image
The official Prometheus Docker image is available on Docker Hub. To use it:
Pull the image:
docker pull prom/prometheus
Run the container:
docker run -d --name prometheus -p 9090:9090 prom/prometheus
You can customize the image by creating your own Dockerfile based on the official image.
Advanced Configurations
For more advanced setups, consider the following options with examples:
1. Using Docker Compose to manage Prometheus
Docker Compose allows you to define and run multi-container Docker applications. Here's an example docker-compose.yml
file that sets up Prometheus:
version: '3'
services:
prometheus:
image: prom/prometheus
volumes:
- ./prometheus.yml:/etc/prometheus/prometheus.yml
ports:
- 9090:9090
command:
- '--config.file=/etc/prometheus/prometheus.yml'
- '--storage.tsdb.path=/prometheus'
- '--web.console.libraries=/usr/share/prometheus/console_libraries'
- '--web.console.templates=/usr/share/prometheus/consoles'
To use this configuration:
- Save it as
docker-compose.yml
- Create a
prometheus.yml
file in the same directory with your Prometheus configuration - Run
docker-compose up -d
in the same directory - Access Prometheus at
http://localhost:9090
This setup allows you to manage Prometheus in a more declarative way, making it easier to maintain and scale your monitoring stack. You can easily add more services to this compose file as your monitoring needs grow.
2. Implementing custom exporters for specific applications
If you have an application that doesn't natively expose Prometheus metrics, you can create a custom exporter. Here's a simple example in Python using the prometheus_client
library:
from prometheus_client import start_http_server, Gauge
import random
import time
# Create a metric to track temperature
temperature = Gauge('room_temperature_celsius', 'Current room temperature in Celsius')
# Function to generate random temperature
def update_temperature():
temperature.set(random.uniform(20.0, 30.0))
if __name__ == '__main__':
# Start up the server to expose the metrics.
start_http_server(8000)
# Generate some random temperatures
while True:
update_temperature()
time.sleep(1)
This exporter creates a custom metric room_temperature_celsius
and exposes it on port 8000. You can then configure Prometheus to scrape this exporter.
3. Setting up federation for large-scale monitoring
Federation allows you to scale Prometheus horizontally. Here's an example configuration for a federated Prometheus setup:
Main Prometheus prometheus.yml
:
scrape_configs:
- job_name: 'federate'
scrape_interval: 15s
honor_labels: true
metrics_path: '/federate'
params:
'match[]':
- '{job="node"}'
static_configs:
- targets:
- 'prometheus-secondary-1:9090'
- 'prometheus-secondary-2:9090'
This configuration allows the main Prometheus instance to collect metrics from two secondary Prometheus instances, focusing on metrics with the job label "node".
4. Integrating with other tools in the Prometheus ecosystem
Using Alertmanager
Alertmanager handles alerts sent by Prometheus server. Here's a basic Alertmanager configuration (alertmanager.yml
):
route:
group_by: ['alertname']
group_wait: 30s
group_interval: 5m
repeat_interval: 1h
receiver: 'email-notifications'
receivers:
- name: 'email-notifications'
email_configs:
- to: 'team@example.com'
To use Alertmanager with Prometheus, add the following to your prometheus.yml
:
alerting:
alertmanagers:
- static_configs:
- targets:
- alertmanager:9093
Using Pushgateway
Pushgateway allows you to push metrics from short-lived jobs. Here's how to push metrics to Pushgateway:
echo "some_metric 3.14" | curl --data-binary @- http://pushgateway:9091/metrics/job/some_job
Then, configure Prometheus to scrape Pushgateway:
scrape_configs:
- job_name: 'pushgateway'
static_configs:
- targets: ['pushgateway:9091']
These advanced configurations allow you to create a more robust and flexible monitoring system, tailored to your specific needs and infrastructure.
Uptrace: A Powerful Alternative to Prometheus
While Prometheus is an excellent tool for monitoring Docker containers, it's worth considering Uptrace as a powerful alternative or complementary solution. Uptrace is an open-source APM (Application Performance Monitoring) platform that can offer several advantages:
Distributed Tracing: Uptrace provides distributed tracing out of the box, allowing you to track requests across multiple services and understand the flow of your applications.
Metrics and Logs Integration: Uptrace combines metrics, logs, and traces in a single platform, providing a more holistic view of your system's performance.
ClickHouse Backend: Uptrace uses ClickHouse as its backend, which allows for fast querying of large datasets and efficient storage of time-series data.
OpenTelemetry Support: Uptrace is built on OpenTelemetry, making it easy to integrate with a wide range of technologies and frameworks.
User-Friendly Interface: Uptrace offers intuitive interface that can make it easier to navigate and understand your monitoring data.
Alerting and Dashboards: Like Prometheus, Uptrace provides robust alerting capabilities and customizable dashboards.
While Prometheus remains a solid choice for many use cases, especially for container-centric environments, Uptrace can provide additional insights and capabilities that may be beneficial for your monitoring needs, particularly in complex, distributed systems and microservices architectures.
Troubleshooting Common Issues
When setting up Prometheus for Docker, you might encounter some issues. Here are solutions to common problems:
Prometheus can't access Docker socket:
- Ensure the Docker socket is correctly mounted
- Check permissions on the Docker socket
Containers not showing up in Prometheus:
- Verify that containers have the correct Prometheus labels
- Check if the container exposes metrics on the specified port
Incorrect metrics:
- Ensure your application is exposing metrics in Prometheus format
- Check if you need to use an exporter for your specific application
High CPU usage:
- Adjust scrape interval in Prometheus configuration
- Optimize your queries and alert rules
Storage issues:
- Configure appropriate storage retention settings
- Consider using remote storage solutions for long-term storage
Conclusion
Setting up Prometheus for Docker provides a powerful monitoring solution for containerized environments. By following this guide, you've learned how to integrate these technologies, implement best practices, and troubleshoot common issues. Remember, effective monitoring is an ongoing process - continue to refine your setup as your needs evolve. Happy monitoring!
You may also be interested in:
- Top 10 Log Analysis Tools in 2024
- Open Source Log Management Tools Comparison
- Advanced Prometheus Querying
- Ingesting Prometheus metrics into Uptrace
FAQ
Can Prometheus monitor non-Docker applications?
Yes, Prometheus can monitor any system or application that exposes metrics in the Prometheus format or has an exporter available.
How does Prometheus handle data storage?
Prometheus stores data locally on disk in its own time-series database format. For long-term storage, it can be integrated with remote storage systems.
What's the recommended hardware for running Prometheus?
Requirements depend on the number of metrics and scrape frequency. Start with at least 2 CPU cores and 4GB RAM, and scale as needed.
Can Prometheus automatically scale with my Docker environment?
Prometheus can automatically discover new Docker containers, but you may need to manually scale Prometheus itself for very large environments.
How do I secure my Prometheus instance?
Use TLS for encryption, implement authentication (e.g., with Nginx reverse proxy), and carefully control access to the Prometheus API.